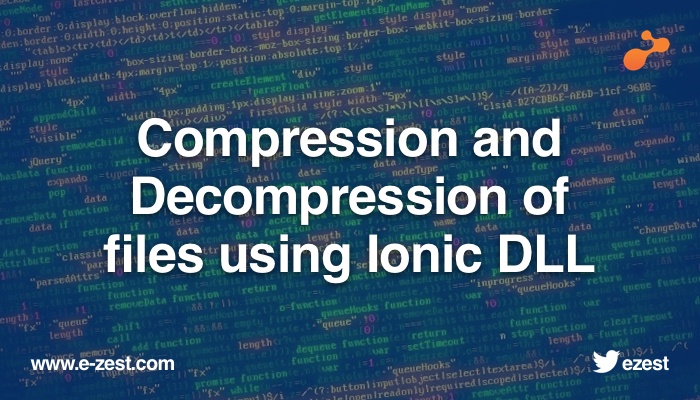
There are many scenarios in which we need to deal with zip and unzip. This will decrease our manual work of zipping and unzipping. Using this, we can make automated processes for the same. .NET has vast improvement in the using System.IO.Compression; namespace. This includes below types to compress and decompress files and streams.
- ZipFile
- ZipArchive
- ZipArchiveEntry
- Zip
First we see for zipping a folder including subfolder or files. Here, we just call the CreateFromDirectory() method of ZipFile.We can also use CreateDirectory(), GetDirectory() methods of ZipArchive class and Open() and Delete() methods of ZipArchiveEntry class
ZipFile.CreateFromDirectory(@"D:\Test\Sample", @"C:\Temp\zipfiles.zip");
Explanation: Note that here we simply provide two parameters to method. First is, what to zip and second is where to put it. By default method considers that level of compression is optimal, we are not providing that here. Other levels are fastest and none.
Other overload for the CreateFromDirectory() method includes two additional options: compression and include root directory.
ZipFile.CreateFromDirectory (@"d:\Test\Sample", @"d:\Temp\zipfiles.zip", CompressionLevel.Optimal, true);
- Unzip
ZipFile.ExractToDirectory (@"d:\Test\MyZippedFolder.zip", @"d:\Test\Sample");
This will unzip the ExamplZip.zip file to the named Sample directory. The complete program is as below.
using System;
using System.IO;
using System.IO.Compression;
namespace ConsoleApplication
{
class Program
{
static void Main(string[] args)
{
string startPath = @"d:\Test\Sample"; //Path of directory to be zipped
string zipPath = @"d:\Test\MyZippedFolder.zip"; //Where the zip file will be placed
string extractPath = @"d:\Test\MyExtractedFile"; // Where the unzip file will be placed
ZipFile.CreateFromDirectory(startPath, zipPath);
MessageBox.Show("Files zipped Successfully");
ZipFile.ExtractToDirectory(zipPath, extractPath);
MessageBox.Show("Files Extracted Successfully");
}
}
}
In the above example we are not able to zip or unzip file size larger than 4GB.
So, we come to alternative solution. We need to use third party dll that is Ionic.zip Dll. It’s free to download.
https://dotnetzip.codeplex.com/ you can download it from here.
Using this we can easily do zip or unzip of file size larger than 4GB.For the same you have to include
Add Ionic.dll to your application references
For example:-
Zip and unzip using Ionic dll
using System.Windows.Forms;
using Ionic.Zip;
using System;
using System.IO;
using System.Windows.Forms;
namespace unzipwindows
{
class Program
{
static void Main(string[] args)
{
ZipFile zip = new ZipFile();
DirectoryInfo f = new DirectoryInfo("c:\\myfolder");
FileInfo[] a = f.GetFiles();
for (int i = 0; i < a.Length; i++)
{
zip.AddFile("d:\\myfolder \\" + a[i].Name, "myfolder"); //second parameter specifies directory or file name in archive
}
zip.Save("d:\\MyZipFile.zip");
MessageBox.Show("Files zipped Successfully");
String TargetDirectory = "c:\\MyZipFile.zip";
String ExtractedDirectory = "d:\\MyExtractedFile";
using (ZipFile zip = ZipFile.Read(TargetDirectory))
{
foreach (ZipEntry d in zip)
{
d.Extract(ExtractedDirectory);
}
MessageBox.Show("Files Extracted Successfully");
}
}
}
}
Hope this will help you!!!